一个简单的自定义栈用C++实现
本文最后更新于:2021年12月21日 晚上
用C++实现一个简单的自定义栈
自定义一个栈(In C++)
在头文件 my_stack.h 中自定义栈
1. 自定义栈的类的数据成员和公有函数:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| #pragma once #include <iostream> using namespace std;
template <typename Type> class Stack { private: Type *stk; int MAXN; int top; public: Stack(int size); ~Stack(); bool empty() const; bool full() const; bool push(Type x); bool pop(); Type get_top() const; int size() const; int Max() const; };
|
2. 函数的定义:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82
| template <typename Type> Stack<Type>::Stack(int size) { MAXN = size; stk = new Type[MAXN]; top = -1; }
template <typename Type> Stack<Type>::~Stack() { delete stk; }
template <typename Type> bool Stack<Type>::empty() const { if (top == -1) { return true; cout << "underflow" << endl; } return false; }
template <typename Type> bool Stack<Type>::full() const { if (top == MAXN - 1) { return true; cout << "overflow" << endl; } return false; }
template <typename Type> bool Stack<Type>::push(Type x) { if (full()) { return false; cout << "overflow" << endl; } stk[++top] = x; return true; }
template <typename Type> bool Stack<Type>::pop() { if (empty()) { return false; cout << "underflow" << endl; } top--; return true; }
template <typename Type> Type Stack<Type>::get_top() const { if (empty()) { return false; cout << "underflow" << endl; } return stk[top]; }
template <typename Type> int Stack<Type>::size() const { return top + 1; }
template <typename Type> int Stack<Type>::Max() const { return this ->MAXN; }
|
在源文件my_stack.cpp中,实现栈的基本操作
1. 创建对象,实现栈的基本操作。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47
| #include <iostream> #include "my_stack.h" using namespace std;
int main() { int n, x, ele, i; enter: cout << "enter max length of stack :" << endl; cin >> n; cout << "--------------------------------" << endl; cout << "enter numbers of elements:" << endl; cin >> x; cout << "--------------------------------" << endl; if (x >= n) { cout << "failed! numbers of elements must less than max length!" << endl << "please enter again!" << endl; goto enter; } Stack<int> s(n); cout << "The max length is : " << s.Max() << endl; cout << "--------------------------------" << endl; cout << "enter your elements:" << endl; for (i = 0; i < x; i++) { cin >> ele; s.push(ele); } cout << "--------------------------------" << endl; cout << "stack size:" << s.size() << endl; cout << "output elements:" << endl;
while (s.empty() != true) { cout << s.get_top() << endl; s.pop(); }
cout << "stack size:" << s.size() << endl; cout << "--------------------------------" << endl;
return 0; }
|
2. 用模板类的时候注意编写的格式
| 模板类外函数定义: template <typename 类型参数> 函数类型 类名<类型参数>::成员函数名(形参表) { …… }
|
运行结果
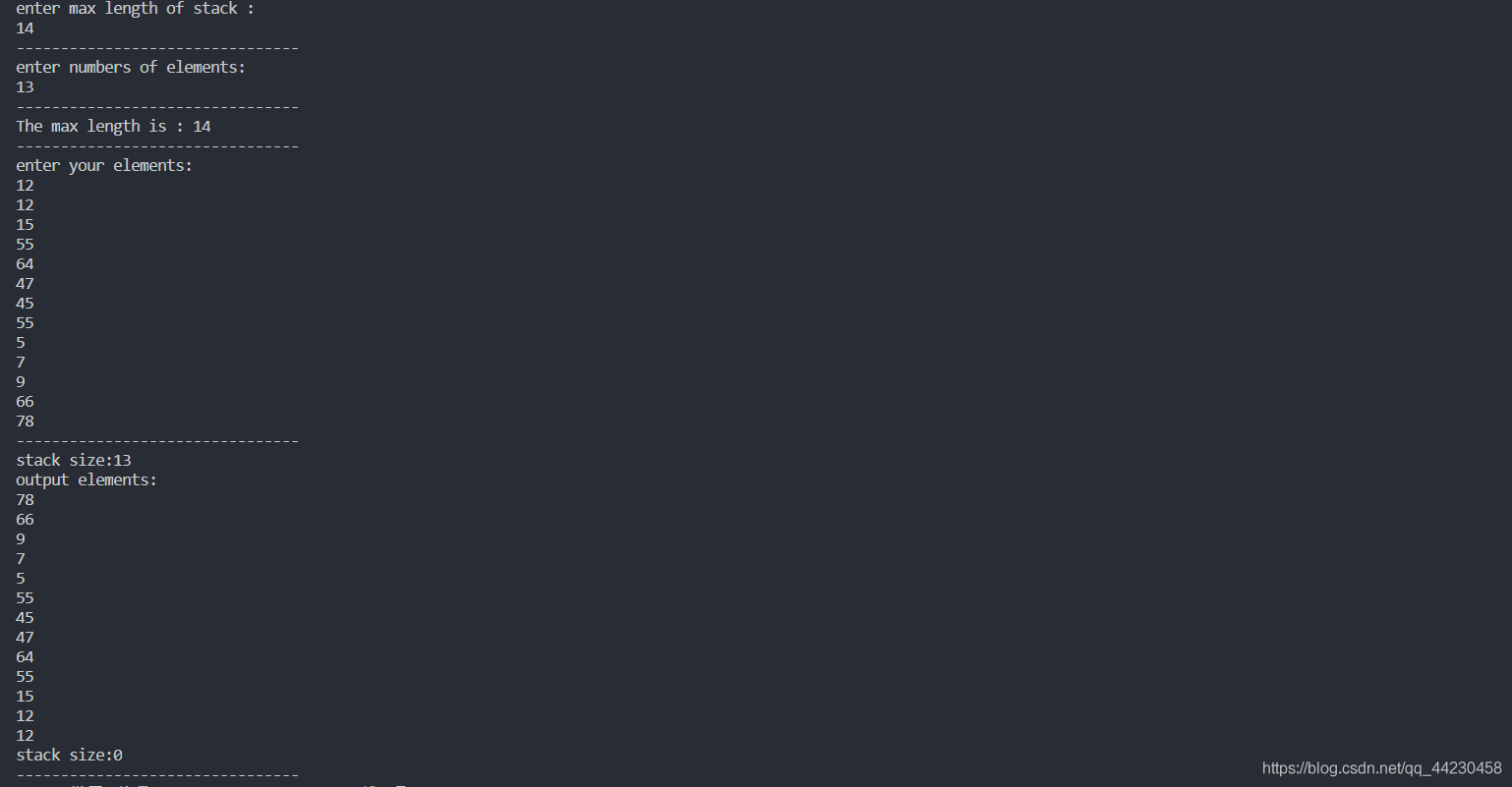